ESP8266 快速参考¶
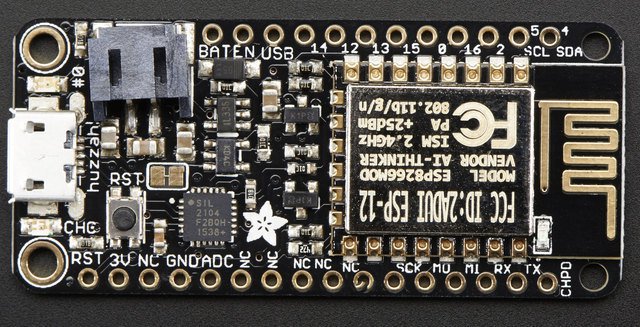
Adafruit Feather HUZZAH 板(图片来源:Adafruit)。
以下是基于 ESP8266 的开发板的快速参考。如果这是您第一次使用该板,请考虑先阅读以下部分:
安装 MicroPython¶
请参阅教程的相应部分:ESP8266 上的 MicroPython 入门。它还包括故障排除小节。
通用板控制¶
MicroPython REPL 位于波特率 115200 的 UART0(GPIO1=TX,GPIO3=RX)上。制表符补全对于找出对象具有哪些方法很有用。粘贴模式 (ctrl-E) 可用于将大量 Python 代码粘贴到 REPL 中。
该 machine
模块:
import machine
machine.freq() # get the current frequency of the CPU
machine.freq(160000000) # set the CPU frequency to 160 MHz
该 esp
模块:
import esp
esp.osdebug(None) # turn off vendor O/S debugging messages
esp.osdebug(0) # redirect vendor O/S debugging messages to UART(0)
联网¶
该 network
模块:
import network
wlan = network.WLAN(network.STA_IF) # create station interface
wlan.active(True) # activate the interface
wlan.scan() # scan for access points
wlan.isconnected() # check if the station is connected to an AP
wlan.connect('essid', 'password') # connect to an AP
wlan.config('mac') # get the interface's MAC address
wlan.ifconfig() # get the interface's IP/netmask/gw/DNS addresses
ap = network.WLAN(network.AP_IF) # create access-point interface
ap.active(True) # activate the interface
ap.config(essid='ESP-AP') # set the ESSID of the access point
连接到本地 WiFi 网络的一个有用功能是:
def do_connect():
import network
wlan = network.WLAN(network.STA_IF)
wlan.active(True)
if not wlan.isconnected():
print('connecting to network...')
wlan.connect('essid', 'password')
while not wlan.isconnected():
pass
print('network config:', wlan.ifconfig())
一旦网络建立起来, socket
模块就可以像往常一样用于创建和使用 TCP/UDP 套接字。
延迟和计时¶
使用 time
模块:
import time
time.sleep(1) # sleep for 1 second
time.sleep_ms(500) # sleep for 500 milliseconds
time.sleep_us(10) # sleep for 10 microseconds
start = time.ticks_ms() # get millisecond counter
delta = time.ticks_diff(time.ticks_ms(), start) # compute time difference
计时器¶
支持虚拟(基于 RTOS)定时器。使用定时器 ID 为 -1的machine.Timer 类:
from machine import Timer
tim = Timer(-1)
tim.init(period=5000, mode=Timer.ONE_SHOT, callback=lambda t:print(1))
tim.init(period=2000, mode=Timer.PERIODIC, callback=lambda t:print(2))
T周期以毫秒为单位。
引脚和 GPIO¶
使用 machine.Pin 类:
from machine import Pin
p0 = Pin(0, Pin.OUT) # create output pin on GPIO0
p0.on() # set pin to "on" (high) level
p0.off() # set pin to "off" (low) level
p0.value(1) # set pin to on/high
p2 = Pin(2, Pin.IN) # create input pin on GPIO2
print(p2.value()) # get value, 0 or 1
p4 = Pin(4, Pin.IN, Pin.PULL_UP) # enable internal pull-up resistor
p5 = Pin(5, Pin.OUT, value=1) # set pin high on creation
可用管脚有:0、1、2、3、4、5、12、13、14、15、16,对应ESP8266芯片实际的GPIO管脚编号。请注意,许多最终用户板使用自己的临时引脚编号(标记为例如 D0、D1、...)。由于 MicroPython 支持不同的板卡和模块,因此选择物理引脚编号作为最小公分母。对于电路板逻辑引脚和物理芯片引脚之间的映射,请查阅您的电路板文档。
注意 Pin(1) 和 Pin(3) 分别是 REPL UART TX 和 RX。另请注意,Pin(16) 是一个特殊的 pin(用于从深度睡眠模式唤醒),可能无法用于更高级别的类,如
Neopixel
.
有一个更高级别的抽象 machine.Signal
或点亮低电平有效 LED 很有用on()
或 value(1)
.
UART(串行总线)¶
参见 machine.UART.
from machine import UART
uart = UART(0, baudrate=9600)
uart.write('hello')
uart.read(5) # read up to 5 bytes
有两个 UART 可用。UART0 位于引脚 1 (TX) 和 3 (RX) 上。UART0 是双向的,默认情况下用于 REPL。UART1 位于引脚 2 (TX) 和 8 (RX) 上,但引脚 8 用于连接闪存芯片,因此 UART1 仅用作 TX。
当 UART0 连接到 REPL 时,UART(0) 上的所有传入字符都会直接进入 stdin,因此 uart.read() 将始终返回 None。如果需要从 UART(0) 读取字符,同时也用于 REPL(或分离、读取、然后重新连接),请使用 sys.stdin.read()。分离后,UART(0) 可用于其他目的。
如果在 REPL 启动时(硬复位或软复位)任何 dupterm 插槽中都没有对象,则 UART(0) 将自动连接。没有这个,在没有 REPL 的情况下恢复电路板的唯一方法是完全擦除和重新刷新(这将安装附加 REPL 的默认 boot.py)。
要将 REPL 与 UART0 分离,请使用:
import uos
uos.dupterm(None, 1)
默认情况下附加 REPL。如果您已将其分离,要重新连接它,请使用:
import uos, machine
uart = machine.UART(0, 115200)
uos.dupterm(uart, 1)
PWM(脉宽调制)¶
PWM 可以在除 Pin(16) 之外的所有引脚上启用。所有通道都有一个频率,范围在 1 到 1000 之间(以 Hz 为单位)。占空比介于 0 和 1023 之间,包括 0 和 1023。
使用 machine.PWM
类:
from machine import Pin, PWM
pwm0 = PWM(Pin(0)) # create PWM object from a pin
pwm0.freq() # get current frequency
pwm0.freq(1000) # set frequency
pwm0.duty() # get current duty cycle
pwm0.duty(200) # set duty cycle
pwm0.deinit() # turn off PWM on the pin
pwm2 = PWM(Pin(2), freq=500, duty=512) # create and configure in one go
ADC(模数转换)¶
ADC 在专用引脚上可用。请注意,ADC 引脚上的输入电压必须介于 0v 和 1.0v 之间。
使用 machine.ADC 类:
from machine import ADC
adc = ADC(0) # create ADC object on ADC pin
adc.read() # read value, 0-1024
软件SPI总线¶
有两个 SPI 驱动程序。一种是在软件中实现的(bit-banging)并在所有引脚上工作,并通过 machine.SoftSPI 类:
from machine import Pin, SoftSPI
# construct an SPI bus on the given pins
# polarity is the idle state of SCK
# phase=0 means sample on the first edge of SCK, phase=1 means the second
spi = SoftSPI(baudrate=100000, polarity=1, phase=0, sck=Pin(0), mosi=Pin(2), miso=Pin(4))
spi.init(baudrate=200000) # set the baudrate
spi.read(10) # read 10 bytes on MISO
spi.read(10, 0xff) # read 10 bytes while outputting 0xff on MOSI
buf = bytearray(50) # create a buffer
spi.readinto(buf) # read into the given buffer (reads 50 bytes in this case)
spi.readinto(buf, 0xff) # read into the given buffer and output 0xff on MOSI
spi.write(b'12345') # write 5 bytes on MOSI
buf = bytearray(4) # create a buffer
spi.write_readinto(b'1234', buf) # write to MOSI and read from MISO into the buffer
spi.write_readinto(buf, buf) # write buf to MOSI and read MISO back into buf
硬件SPI总线¶
硬件 SPI 速度更快(高达 80Mhz),但仅适用于以下引脚:
MISO
GPIO12, MOSI
GPIO13 和 SCK
GPIO14。除了构造函数和 init 的引脚参数(因为这些是固定的)之外,它具有与上面的 bitbanging SPI 类相同的方法:
from machine import Pin, SPI
hspi = SPI(1, baudrate=80000000, polarity=0, phase=0)
(SPI(0)
用于 FlashROM,用户不可用。)
I2C总线¶
I2C 驱动程序在软件中实现并在所有引脚上工作, 并通过 machine.I2C 类(它是 的别名machine.SoftI2C):
from machine import Pin, I2C
# construct an I2C bus
i2c = I2C(scl=Pin(5), sda=Pin(4), freq=100000)
i2c.readfrom(0x3a, 4) # read 4 bytes from slave device with address 0x3a
i2c.writeto(0x3a, '12') # write '12' to slave device with address 0x3a
buf = bytearray(10) # create a buffer with 10 bytes
i2c.writeto(0x3a, buf) # write the given buffer to the slave
实时时钟 (RTC)¶
参见 machine.RTC
from machine import RTC
rtc = RTC()
rtc.datetime((2017, 8, 23, 1, 12, 48, 0, 0)) # set a specific date and time
rtc.datetime() # get date and time
# synchronize with ntp
# need to be connected to wifi
import ntptime
ntptime.settime() # set the rtc datetime from the remote server
rtc.datetime() # get the date and time in UTC
笔记
并非所有方法都已实现: RTC.now()
, RTC.irq(handler=*)
(使用自定义处理程序),RTC.init()
和 RTC.deinit()
并且
当前不受支持。
深度睡眠模式¶
将 GPIO16 连接到复位引脚(HUZZAH 上的 RST)。然后可以使用以下代码进行睡眠、唤醒和检查复位原因:
import machine
# configure RTC.ALARM0 to be able to wake the device
rtc = machine.RTC()
rtc.irq(trigger=rtc.ALARM0, wake=machine.DEEPSLEEP)
# check if the device woke from a deep sleep
if machine.reset_cause() == machine.DEEPSLEEP_RESET:
print('woke from a deep sleep')
# set RTC.ALARM0 to fire after 10 seconds (waking the device)
rtc.alarm(rtc.ALARM0, 10000)
# put the device to sleep
machine.deepsleep()
单线驱动¶
OneWire 驱动程序在软件中实现并适用于所有引脚:
from machine import Pin
import onewire
ow = onewire.OneWire(Pin(12)) # create a OneWire bus on GPIO12
ow.scan() # return a list of devices on the bus
ow.reset() # reset the bus
ow.readbyte() # read a byte
ow.writebyte(0x12) # write a byte on the bus
ow.write('123') # write bytes on the bus
ow.select_rom(b'12345678') # select a specific device by its ROM code
DS18S20 和 DS18B20 设备有一个特定的驱动程序:
import time, ds18x20
ds = ds18x20.DS18X20(ow)
roms = ds.scan()
ds.convert_temp()
time.sleep_ms(750)
for rom in roms:
print(ds.read_temp(rom))
一定要在数据线上放一个4.7k的上拉电阻。请注意, convert_temp()
每次要对温度进行采样时都必须调用该方法。
NeoPixel 驱动程序¶
使用neopixel
模块:
from machine import Pin
from neopixel import NeoPixel
pin = Pin(0, Pin.OUT) # set GPIO0 to output to drive NeoPixels
np = NeoPixel(pin, 8) # create NeoPixel driver on GPIO0 for 8 pixels
np[0] = (255, 255, 255) # set the first pixel to white
np.write() # write data to all pixels
r, g, b = np[0] # get first pixel colour
For low-level driving of a NeoPixel:
import esp
esp.neopixel_write(pin, grb_buf, is800khz)
警告
默认 NeoPixel
配置为控制更流行的800kHz 单位。timing=0
在构造NeoPixel
object.对象时, 可以使用替代时序来控制其他(通常为 400kHz)设备。
APA102驱动¶
使用apa102
模块:
from machine import Pin
from apa102 import APA102
clock = Pin(14, Pin.OUT) # set GPIO14 to output to drive the clock
data = Pin(13, Pin.OUT) # set GPIO13 to output to drive the data
apa = APA102(clock, data, 8) # create APA102 driver on the clock and the data pin for 8 pixels
apa[0] = (255, 255, 255, 31) # set the first pixel to white with a maximum brightness of 31
apa.write() # write data to all pixels
r, g, b, brightness = apa[0] # get first pixel colour
对于 APA102 的低电平驱动:
import esp
esp.apa102_write(clock_pin, data_pin, rgbi_buf)
DHT驱动程序¶
DHT 驱动程序在软件中实现并适用于所有引脚:
import dht
import machine
d = dht.DHT11(machine.Pin(4))
d.measure()
d.temperature() # eg. 23 (°C)
d.humidity() # eg. 41 (% RH)
d = dht.DHT22(machine.Pin(4))
d.measure()
d.temperature() # eg. 23.6 (°C)
d.humidity() # eg. 41.3 (% RH)
SSD1306驱动¶
用于 SSD1306 单色 OLED 显示器的驱动程序。请参阅 显示器教程SSD1306 OLED .
from machine import Pin, I2C
import ssd1306
i2c = I2C(scl=Pin(5), sda=Pin(4), freq=100000)
display = ssd1306.SSD1306_I2C(128, 64, i2c)
display.text('Hello World', 0, 0, 1)
display.show()
WebREPL(网络浏览器交互提示)¶
WebREPL(基于 WebSockets 的 REPL,可通过网络浏览器访问)是 ESP8266 端口中可用的实验性功能https://github.com/micropython/webrepl (托管版本可在 http://micropython.org/webrepl), 并通过执行来配置它:
import webrepl_setup
并按照屏幕上的说明进行操作。重启后就可以连接了。如果您在启动时禁用了自动启动,您可以使用以下命令按需运行配置的守护程序:
import webrepl
webrepl.start()
支持使用 WebREPL 的方法是连接到 ESP8266 接入点,但如果 STA 接口处于活动状态,守护程序也会在 STA 接口上启动,因此如果您的路由器设置并正常工作,您也可以在连接到普通 Internet 时使用 WebREPL接入点(如果您遇到任何问题,请使用 ESP8266 AP 连接方法)
除了终端/命令提示符访问,WebREPL 还提供文件传输(上传和下载)。Web 客户端具有对应功能的按钮,或者您可以使用 webrepl_cli.py
上面存储库中的命令行客户端。
请参阅 MicroPython 论坛,了解其他社区支持的将文件传输到 ESP8266 的替代方法。